Ember Handlebar helpers and img objects
Today I spend about an hour figuring out how I could return jQuery generated img html object from an (ember) Handlebars helper and have it rendered as markup in the document. O boy what a battle… I was a bit surprised that this wasn’t easier and/or better documented.
In the end I decided to get the node as a string and return it from the helper as a Handlebars.SafeString
. You might not know or realize this, but it’s actually not that easy when you hold a HTMLImageElement to get the text representation of it. In my case I was using the Cloudinary jQuery plugin to get an image from my CDN, the .image()
method here returns a jQuery image node.
The example:
1
2
3
4
5
6
7
Ember.Handlebars.helper('gallery-image', function(gallery_image) {
var image = $.cloudinary.image(
gallery_image.get('asset'),
{ format: 'png', crop: 'fill', width: 150, height: 100 } );
var html = $("<div />").append(image).html();
return new Ember.Handlebars.SafeString(html);
});
We start of here by creating the helper named gallery-image
. The argument to the helper is a model record containing an asset field which contains the public_id of the image on the Cloudinary CDN.
So the line that does the trick here is:
1
var html = $("<div />").append(image).html();
This is the only way to get the string version of the img tag. Simply calling new String(image) wouldn’t work as then you get the string representation of the Javascript Image object, which of course isn’t what were looking for. What happens here is that we create a new node, the div, and append to that the Image object after that we use the jQuery html()
function to get the innerHTML
of the div
. The last step is to return from the helper using Ember.Handlebars.SafeString
so that it’s indeed rendered as html and not escaped. This is the only way I could figure out how to do this. Surely I’m not the first with this requirement and hopefully I’m missing something completely obvious and there’s a much neater way of doing this.
I should point out that I realize that I could have written a template for this and then rendered that out in my helper, but seriously, having to write a template for such a trivial use case is like going duck hunting with a tank….
Suggestions, tips or flames? Please leave a comment. ♯web development ♯cloudinary ♯handlebars ♯front-end ♯javascript ♯ember
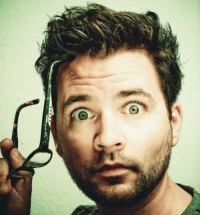